概要
- componentを画面に表示しようとしたらProps設定に次のエラーが発生しました。
- 結果としてはPropsの使い方の問題だったので解決するまでの対応を整理しました。

開発環境
- React 19.0.0-rc-69d4b800-20241021
- Next.js 15.0.1
- VS Code
エラー内容
- 画面にcomponentを表示だけの簡単な処理ですが次のエラーが発生しました。
- それに開発環境(VS Code)上では次のエラーが発生しますが、
画面ではエラーは発生せず何も表示されないだけでした。 - 以前対応したエラーとはエラー内容は似ているようでしたが違うエラーのようです。
[React / Next.js / Error] Type ‘XXX’ is not assignable to type ‘XXX’. のエラー

- そして次がソースコードです。
src\app\test\TestProps.tsx
export type TestProps = {
test1 : string
test2 : number
test3 : boolean
}
- booleanはそのままでは表示されないので少し工夫が必要です。
src\app\test\component.tsx
import { TestProps } from "./TestProps"
export default function component(props : TestProps) {
return (
<>
<div>{props.test1}</div>
<div>{props.test2}</div>
<div>{props.test3&&"true"}</div>
</>
);
}
src\app\test\page.tsx
import { TestProps } from "./TestProps"
import Component from './component'
export default function Home() {
const testProps : TestProps = {
test1 : "test",
test2 : 1,
test3 : true
}
return (
<Component testProps={testProps}/>
);
}
参考
- [React / Next.js / Error] Type ‘XXX’ is not assignable to type ‘XXX’. のエラー
- 「Type ‘string | undefined’ is not assignable to type ‘string’. Type ‘undefined’ is not assignable to type ‘string’」の解決方法
- TypeScript の困った型エラーを解消したい
- 【TypeScript】Reactのコンポーネントに値を渡すとIntrinsicAttributesに代入できないと怒られる
原因
- Props、Componentまでは問題ないですが、
page側で利用するところでの知識不足でした。
対応
方法1. Propsの各項目を分けて設定する
- 各データを「データ名 = {データ}」で分けて設定します。
- 今回はTestPropsタイプ testPropsのtest1、test2、test3を一つずつ設定しました。
src\app\test\page.tsx
import { TestProps } from "./TestProps"
import Component from './component'
export default function Home() {
const testProps : TestProps = {
test1 : "test",
test2 : 1,
test3 : true
}
// 1. 各データを「データ名 = {データ}」で分けて設定します。
return (
<Component
test1={testProps.test1}
test2={testProps.test2}
test3={testProps.test3}/>
);
}
- それでエラーが解決されまして表示できるようになりました。
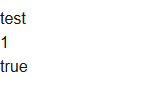
方法2. {…}構文を利用する
- {…} 構文を利用してtestPropsの各データを一つずつ分けて設定します。
src\app\test\component.tsx
import { TestProps } from "./TestProps"
import Component from './component'
export default function Home() {
const testProps : TestProps = {
test1 : "test",
test2 : 1,
test3 : true
}
// 1. {...}構文を利用してtestPropsの各データを一つずつ分けて設定します。
return (
<Component {...testProps}/>
);
}
- それでエラーが解決されまして表示できるようになります。
まとめ
- Propsを設定してcomponentを利用する際に、
Type ‘XXX’ is not assignable to type ‘IntrinsicAttributes & XXX’. のエラーが
発生した場合の対応をまとめました。 - 方法1, 2どちらでも解決はできますが
- Propsタイプのデータが多かったり、
- typeの変更時には変更に合わせて対応が発生する
- などを考えると個人的には方法2がおすすめです。